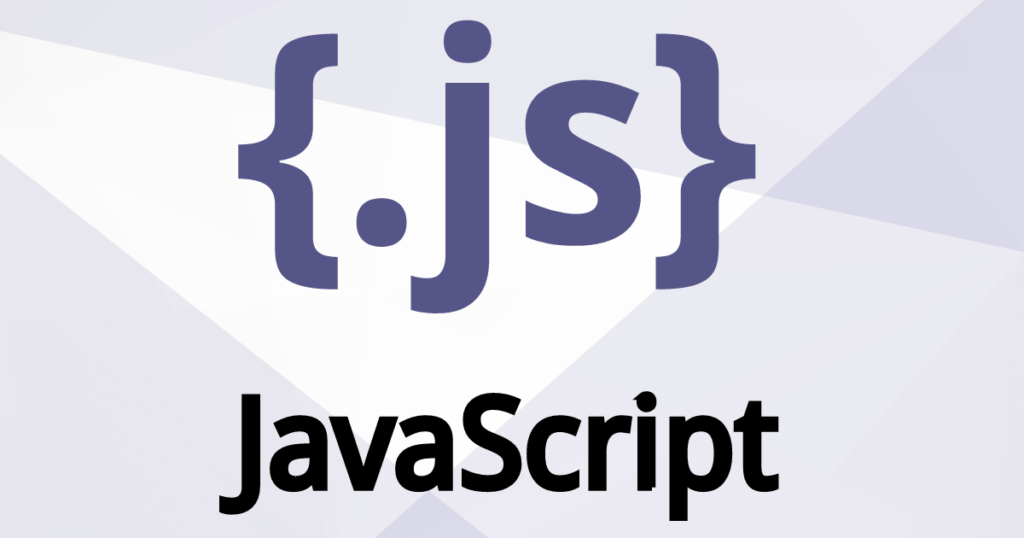
In the fast-paced world of web development, front-end mastery is key. This blog will outline a strategic learning path with a detailed syllabus and recommend top online courses to help you build visually appealing and user-friendly websites.
Introduction
Front-end development involves creating the visual and interactive aspects of a website or web application. This field focuses on what users see and interact with directly, encompassing everything from layout and design to buttons and navigation menus. Front-end developers use a combination of technologies, primarily HTML, CSS, and JavaScript, to build websites that are both functional and aesthetically pleasing. HTML gives the layout to a web page, CSS gives looks and feels, whereas JavaScript provides functionality to a web page whenever any event like a click or scroll occurs.
To enhance and streamline development, various frameworks and libraries are used. For CSS, popular frameworks include Bootstrap and Tailwind CSS, which provide pre-designed components and utilities. In 2024, Tailwind CSS is the most popular framework that I recommend to learn. For JavaScript, frameworks, and libraries like ReactJS, Angular, and Vue.js are commonly used to build dynamic and interactive user interfaces. But, ReactJS is in highest demand nowadays because It is easy to learn and also it has maximum job opportunities. Along with JavaScript, TypeScript is also in demand as it makes development effective because of its static typing and its ability to find errors during development. Additionally, CSS preprocessors like SASS allow developers to write more maintainable and scalable CSS.
Learning a framework will give improve your web development career as it makes web development very fast, but, don’t become a framework guy. Learning basics is essential. Basics are helpful in every stage of development. It helps you build logic, identify errors, and improve problem-solving skills. I believe that whoever has a strong command over the basics of HTML, CSS, and JavaScript and is able to make a complete project with the help of these technologies, will not be afraid of any change in technologies in the future because any framework or any update is over the top of basic programming concepts.
The front end interacts with the back end using APIs (Application Programming Interfaces), which can be third-party services or custom-built by developers. These APIs enable the front end to request and receive data from the back end, which then serves the response in the form of resources like data or files.
Link To Familiar With Web Development: Click Here
Front-End Developer Roadmap
- HTML (HyperText Markup Language)
- CSS (Cascading Style Sheet)
- JavaScript
- TypeScript
- Tailwind CSS
- SASS (A CSS pre-processor)
- ReactJs (A JavaScript framework)
- NextJs (A framework to build React-based applications for Server Side Rendering and static website generation)
Detailed Roadmap For Front-End Development
HTML (HyperText Markup Language)
HTML stands for HyperText Markup Language. It is the standard markup language used for creating and structuring web pages and web applications. HTML provides a set of elements or tags that define the structure and content of a web page. These elements are used to enclose different parts of content, such as headings, paragraphs, images, links, forms, and more. We create a layout of a web page with the help of HTML. HTML is the first step towards front-end development.
It is a combination of two definitions–> (HyperText + Markup Language)
HyperText: It refers to the pieces of text that can link to other documents on the website. For example, we use anchor tags (<a>) to link to other websites or a web page.
Markup Language: Markup with HTML is done through HTML tags. A markup language is a text-encoding system that applies the structure and formatting of a document and potentially the relationship between the parts.
Syllabus
- What is HTML?
- How does HTML render on the website?
- What is a Meta tag?
- How CSS or JavaScript files are linked to an HTML file?
- How to use Chrome Developer Tool to manipulate a website.
- Tags: <html>, <title>, <body>, <nav>, <main>, <footer>, <div>
- Heading tags: <h1>, <h2>, <h3>, <h4>, <h5>, <h6>
- Paragraph tag: <p>
- Self Closing Tags: <br/>, <hr/>
- List tags: <ul> for Unordered List, <ol> for unordered List
- Anchor tag: <a>
- Image tag: <img>
- Attributes: href, src, target
- Text Formatting Tags: <strong>, <em>
- File Paths: (Absolute and Relative Paths)
- Table tags: <table>, <th>, <tr>, <td>, <colspan>, <rowspan>
- Form tags: <form>, <method>, <action>, <input>, <label>, <submit>, <button>, <name>, <value>, <select>, <option>, <textarea>
- Multimedia: <audio>, <video>
You can learn HTML from W3Schools:- Click Here
Official Documentation: Click Here
CSS (Cascading Style Sheets)
Cascading Style Sheets is a style sheet language used for specifying the presentation and styling of a document written in a markup language such as HTML.
Syllabus
- Adding CSS in HTML file: Inline CSS, Internal CSS, External CSS
- CSS Selectors: Element Selector, Class Selector, ID Selector, Attribute Selector, Universal Selector
- CSS Properties
- colors: color, background color, opacity
- Typography: font size, font weight, font family, line height, text-align, text-decoration
- Units: px, pt, em, rem, vh, %
- CSS Box Model: Margin, Padding, Border, Content, Box height and width
- Content Division Element: <div>
- The Cascade: Specificity and Inheritance
- Combine CSS Selectors
- CSS Positions: Static positioning, Relative positioning, Absolute positioning, Fixed positioning
- Display property: Block, Inline, and Inline-Block
- CSS Float (float: left, float: right)
- Flexbox: display, flex-direction, flex, flex-basis, flex-width, max-width, min-width, flex-wrap, justify-content, align-items
- CSS Grid: display, grid-template-rows, grid-template-columns, gap, grid-area
- Media Query: @media, max-width, min-width
Free Resource To Learn CSS: W3Schools
Paid Resource for comprehensive and good projects: Build Responsive Real-World Websites with HTML and CSS By Jonas Schmedtmann
Official Documentation: Click Here
Project Ideas
- Personal Portfolio Website to showcase your skills and projects.
- Landing Page for an e-commerce website.
- Blog Layout
- Photo Gallery to showcase electronic gadgets
- Interactive Resume
JavaScript
JavaScript is a lightweight, interpreted, object-oriented programming language with first-class functions, primarily known as the scripting language for web pages, but it’s also used in many non-browser environments.
Syllabus
- Fundamentals
- Data Types, Variables (let, const, var), Operators, String, Template, Literals, Taking Decisions (if-else), Looping (for loop, while loop, do while loop), Type Conversion & Coercion, Truthy & Falsy Values, Equality Operators (=== & ==), switch, ternary operator
- Functions
- Function Declaration, Function Expression, Arrow Function
- Objects (Structuring And Destructuring of objects)
- DOM Manipulation
- Scoping (global scope, function scope, block scope)
- Hoisting
- The this keyword
- Arrays
- Short-Circuiting
- Optional Chaining
- Sets, Maps
- Advanced DOM
- Asynchronous JavaScript: Promises, Async/Await
- Axios for fetching data from third-party API
Udemy Course For JavaScript: The Complete JavaScript Course 2024: From Zero to Expert! By Jonas Schmedtmann
Official Documentation: Click Here
Projects
Use HTML and CSS along with JavaScript to design your applications
- Dice Game (HTML, CSS, JavaScript
- Weather Application
- To-Do List Application
- Budget Tracker
- Fitness Tracker
- Game Site by fetching data from open-source API
- Quiz Application
TypeScript
TypeScript is a typed superset of JavaScript that compiles to plain JavaScript. TypeScript adds optional static types, classes, and modules to JavaScript, enabling developers to build large-scale applications and write code that is easier to understand and maintain.
Syllabus
- Types In TypeScript
- Basic Types: Number, String, Boolean, Array, Tuple, Enum, Any, Void, Null And Undefined, Never, Object
- Type Annotations And Type Inference
- Union And Intersection Types
- Literal Types: String Literal Types
- Type Aliases
- Type Assertions
- Interfaces: Defining, extending, read-only properties, optional properties, implementing, generics with interfaces
- Classes: methods, properties, constructors, inheritance, access modifiers
- Functions: optional parameters, default parameters, rest parameters
- Generics
- Namespaces vs Modules
Free Resource For Complete TypeScript:- Click Here
React
React is a JavaScript library for building user interfaces, developed by Facebook and the open-source community. It lets you compose complex UIs from small and isolated pieces of code called “components”.
Syllabus
- Basics
- Key Features of React
- Virtual DOM
- What are React Components?
- Functional components and Class components
- What is a single-page application (SPA)?
- JSX
- Role Of JSX in React?
- What is Babel?
- Role of Fragment in JSX?
- Spread Operator in JSX?
- Conditional Rendering
- What is Transpiler?
- How React Provides Reusability and Composition
- State and State Management
- Props
- Folder Structure
- Passing data between components
- Prop Drilling
- Routing
- React Hooks: useState, useEffect, useContext, useReducer, useCallback, useMemo, useRef, useLayoutEffect
- Life-Cycle Methods
- Code Splitting
- Axios Library
- Redux
- Forms: React-hook-forms, zod
- Data fetching: fetch API, axios
Projects For React:
You can build the same projects that you build with HTML, CSS and JavaScript.
SASS
Sass is a preprocessor scripting language that is interpreted or compiled into Cascading Style Sheets (CSS). It introduces features like variables, nested rules, and mixins, which enhance the capabilities of plain CSS and make styling more maintainable and scalable for large projects.
Syllabus
- Basics
- variables, variable scope
- nesting selectors, nesting properties
- partials and imports
- SASS features
- mixins, @include to apply mixins
- inheritance (extending styles with @extend, placeholder selectors (%))
- Functions
- Built-in SASS functions
- Writing Custom Functions
- Control Directives and Expressions
- Conditional Statements (@if, @else-if, @else)
- Loops (@for, @each, @while)
- Using @mixin, and @include within loops and conditionals
- Math operations
- Color functions
Udemy Course For Advanced CSS And SASS: Advanced CSS and Sass: Flexbox, Grid, Animations and More! By Jonas Schmedtmann
Tailwind CSS
Tailwind CSS is a utility-first CSS framework for rapidly building custom user interfaces. It provides a comprehensive set of utility classes that can be composed to create any design directly in your HTML.
Syllabus
- Utility First Approach
- Customization using configuration file tailwind.config.js
- Typography, layout, spacing, and color utilities
- Flexbox and Grid Utilities
- Plugins and Extensions
- JIT Mode and PurgeCSS
- Framework Integration (React)
- Performance Optimization
Udemy Course For Tailwind CSS: Tailwind CSS From Scratch | Learn By Building Projects By Brad Traversy
Automated Testing
Jest and ViTest are the most popular automation testing frameworks. But, ViTest is new and gaining more popularity in web development.
- Basics of ViTest
- Initial Setup and Configuration
- Understanding testing environment
- Matchers in ViTest
- Common matchers:
toBe
,toEqual
,toContain
- Truthiness matchers:
toBeTruthy
,toBeFalsy
- Null and undefined matchers:
toBeNull
,toBeUndefined
,toBeDefined
- String and object matchers:
toMatch
,toMatchObject
- Property and length matchers:
toHaveProperty
,toHaveLength
- Common matchers:
- Mocking in Vitest
- Mocking functions and modules
- Using timers in mock scenarios
- Best practices for creating mocks and spies
- Handling Asynchronous Code
- Testing with async/await
- Managing promises in test cases
- Ensuring Asynchronous Code Executes correctly
- Code Coverage in Vitest
- Collecting code coverage data
- Generating and interpreting coverage reports
- Configuring coverage thresholds and ensuring comprehensive testing
- Testing React Components
- Using React Testing Library with Vitest
- Query methods:
get
,query
,find
- Matchers for React components:
toBeChecked
,toBeDisabled
,toBeInTheDocument
- Attribute and content matchers:
toHaveAttribute
,toHaveTextContent
- Simulating user events with
user-event
- Mocking APIs with Mock Service Worker (MSW)
- Setting up MSW for API mocking
- Creating mock service handlers
- Testing API interactions and ensuring reliable responses
Udemy Course For Automation Testing: React Testing Library with Jest / Vitest by Bonnie Schulkin
NextJs
Next.js is a React framework that enables functionality such as server-side rendering and generating static websites for React based web applications. It allows for efficient and optimized React application development, providing features like automatic code splitting, route pre-fetching, and built-in CSS and Sass support.
Syllabus
- Getting Started
- Setting up a Next.js project
- Project structure and basics of file-based routing
- Data Fetching
- Static Generation (SSG) with
getStaticProps
andgetStaticPaths
- Server-Side Rendering (SSR) with
getServerSideProps
- Client-side fetching with SWR
- Static Generation (SSG) with
- Routing and Navigation
- File-based routing and dynamic routes
- Navigation between pages using the
Link
component
- Styling
- Using CSS modules for scoped styles
- Global CSS and CSS-in-JS libraries integration
- Optimization
- Image optimization with the
Image
component - Performance optimization techniques like code splitting and lazy loading
- Image optimization with the
- Deployment and Environment
- Deployment to Vercel and other hosting platforms
- Configuration management and environment variables
- Advanced Features
- API Routes for creating serverless endpoints
- Authentication with NextAuth.js or other methods
- TypeScript Integration
- Setting up TypeScript in Next.js projects
- Typing components and data fetching functions
- SEO and Accessibility
- Managing SEO with the
Head
component - Ensuring accessibility best practices
- Managing SEO with the
- Testing and Debugging
- Setting up Jest and React Testing Library for testing
- Debugging techniques and error handling
- Customization and Extensibility
- Customizing the
_document.js
and_app.js
files - Adding middleware and custom server logic
- Customizing the
Free YouTube Course For NextJs: Click Here
Conclusion
Front-end development focuses on creating the visual and interactive aspects of websites using HTML, CSS, and JavaScript. Mastering these basics is crucial before diving into popular frameworks like ReactJS and tools like Tailwind CSS, which enhance development efficiency.
A solid foundation in core technologies allows developers to adapt easily to new trends. Following a structured learning roadmap—from HTML and CSS basics to advanced JavaScript, TypeScript, and frameworks like React and Next.js—ensures comprehensive skill development.
Incorporating tools like SASS for scalable CSS and ViTest for automated testing ensures robust, maintainable code. Understanding API integration for back-end communication further extends functionality.
By building on these essentials and staying updated, you can excel in the dynamic field of front-end development.
2 thoughts on “Front-End Development”